Okay, so I’ve been messing around with this thing called Retrofit for a while now, and let me tell you, it’s been quite the journey. I wanted to share my experience, and hopefully, it’ll be helpful to some of you out there.
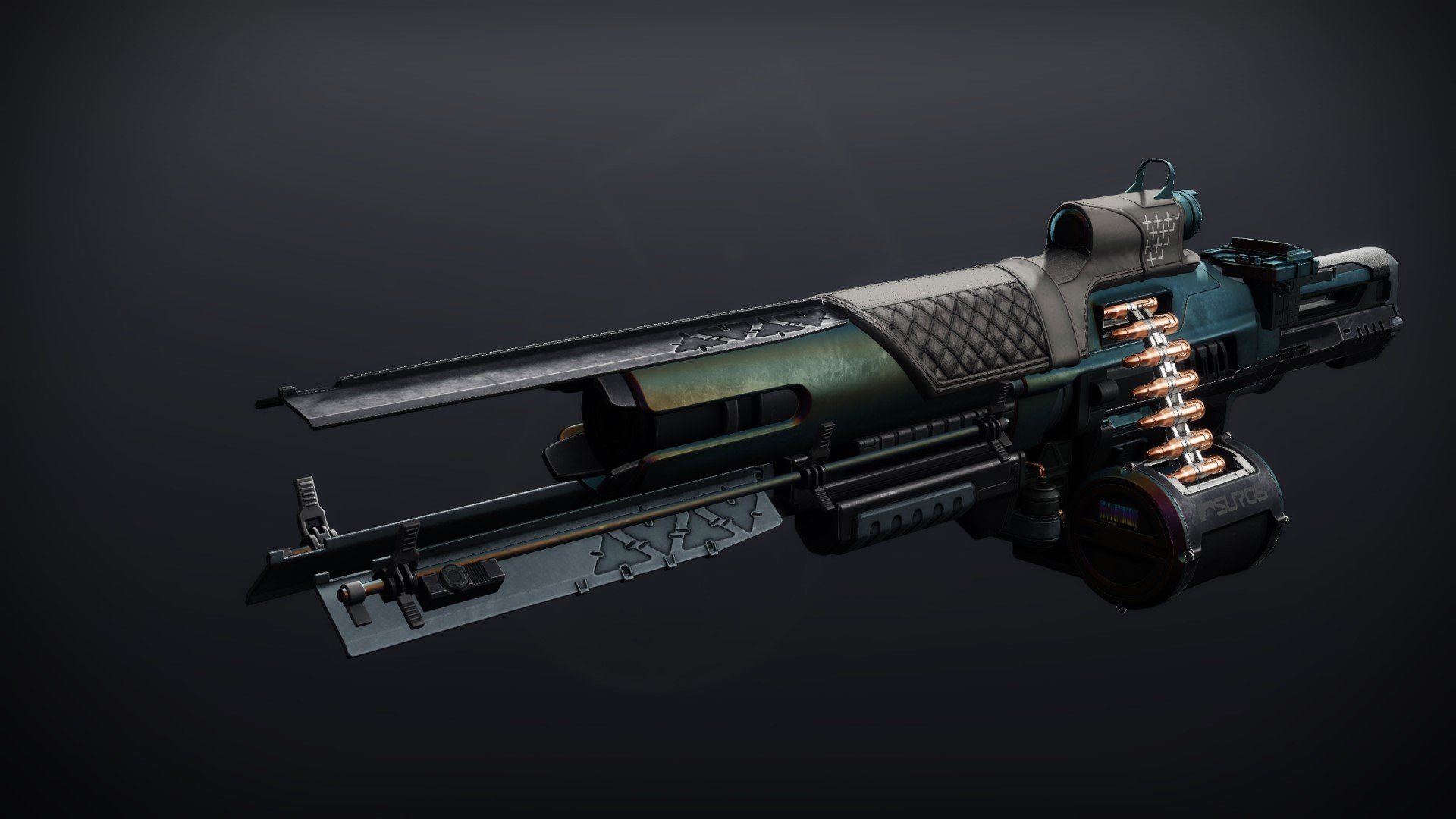
Getting Started
First off, I had to get this Retrofit thing into my project. I added the dependency to my build file. I went with the latest version, figuring it would be the best, right? Also, I needed a converter to handle the JSON responses from the server, so I added the Gson converter too.
- Added Retrofit dependency
- Added Gson converter dependency
Setting Up the Interface
Next, I created an interface to define my API endpoints. This is where you specify the HTTP methods (like GET, POST, etc.), the URLs, and the parameters. I started simple, with just one GET request to fetch some data.
Creating the Retrofit Instance
With the interface ready, I had to set up a Retrofit instance. This involved creating a Retrofit object and configuring it with the base URL of my API and the converter factory I wanted to use. I picked Gson because that’s what I was familiar with.
I remember spending a good chunk of time figuring out the correct base URL. Turns out, I had a trailing slash missing, which caused some headaches. Lesson learned: double-check your URLs!
Making the First Call
Time for the real test! I used the Retrofit instance to create an implementation of my API interface. Then, I called the method I defined earlier to make the GET request. I had to handle the response in a callback, which felt a bit weird at first, but I got used to it.
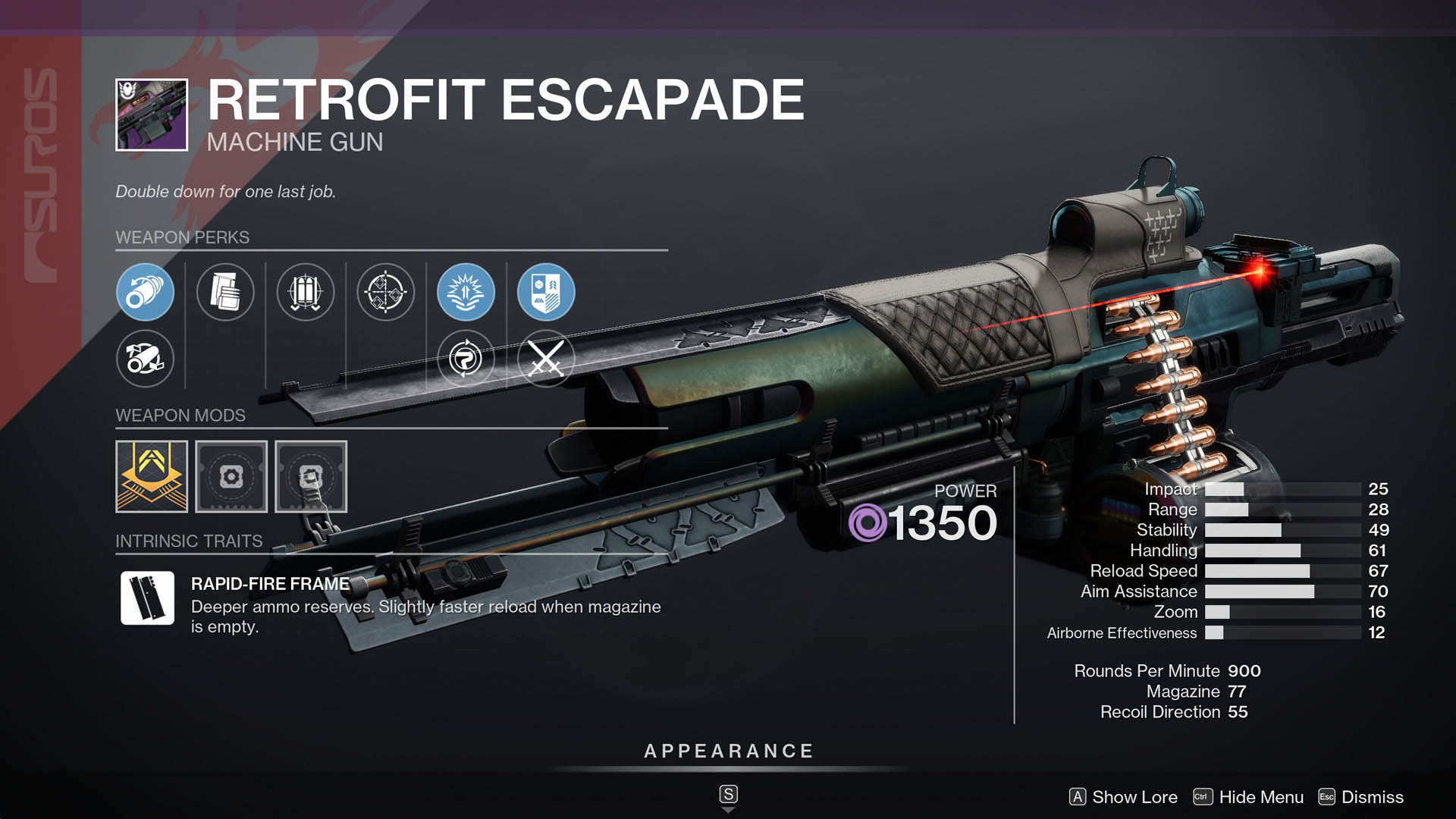
Handling the Response
The callback gave me two options: success and failure. In the success case, I got the data I was expecting, parsed by Gson into a nice Java object. In the failure case, I had to deal with errors. This involved checking the status code and the error message. Honestly, the error handling part wasn’t super fun, but it’s crucial.
Adding More Features
Once I got the basic GET request working, I started adding more complex features. I experimented with POST requests, sending data to the server. Then I added query parameters, headers, and even dynamic URLs. It was a lot of trial and error, but I gradually figured it out.
I also tried a few different converters. At first, I had some trouble with a converter, but Gson worked fine for me.
What I Learned
Retrofit makes it pretty easy to interact with REST APIs. The code is cleaner, more readable than manually making HTTP requests. It’s definitely worth checking out. It will simplify your code and make your life easier. You just need to make sure to handle the response correctly, especially the errors. You know, it is not always successful.
So that’s my Retrofit escapade. It was a learning experience, for sure, but I’m glad I went through it. I hope my rambling has been somewhat useful. Go forth and code!
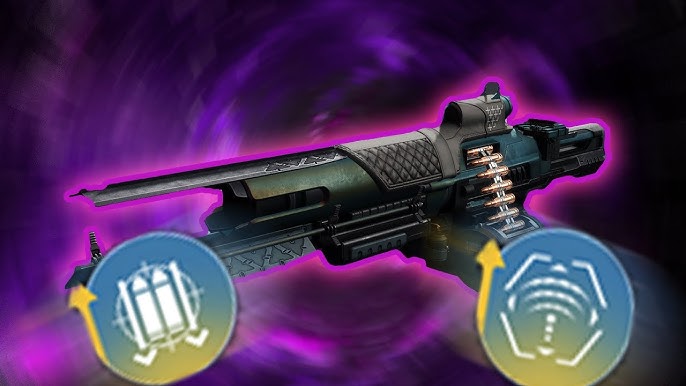